Java Abstract Class Interview Questions and Answers
Java Abstract classes are particularly useful when there’s a need to model complex relationships between objects or adhere to specific design patterns. They enable developers to establish a contract for derived classes, ensuring consistency and conformity across all implementations. A class that contains the abstract keyword in its declaration is an abstract class, and it may have both abstract and non-abstract methods. Abstraction in Java hides complex code implementations for viewers and offers them only necessary information.
There are situations in which you will want to define a superclass that declares the structure of a given abstraction without providing a complete implementation of every method. That is, sometimes you will want to create a superclass that only defines a generalized form that will be shared by all of its subclasses, leaving it to each subclass to fill in the details. Such a class determines the nature of the methods that the subclasses must implement. One way this situation can occur is when a superclass is unable to create a meaningful implementation for a method.
In the realm of object-oriented programming (OOP), abstract classes play a pivotal role in establishing structure and promoting code reusability. An abstract class serves as a blueprint for other classes, defining common properties and methods that can be inherited by its subclasses. However, an abstract class cannot be instantiated directly; it is designed to provide a base for other classes to build upon.
TechShitanshu has prepared a list of the top java Abstract Class interview questions and answers that are frequently asked in the interview. It is going to help you to crack the java interview questions and answers to get your dream job.
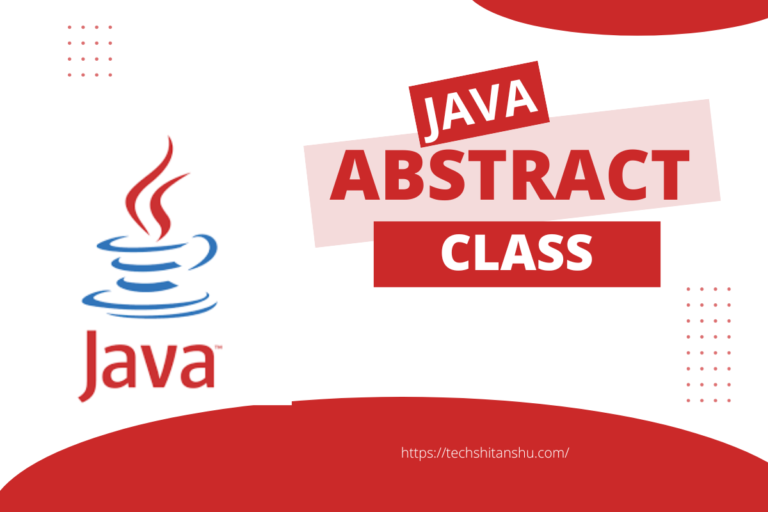
Java abstract class interview questions
1. What is Abstraction in Java?
Abstraction in Java is a technique by which we can hide the data that is not required to users. It hides all unwanted data so that users can work only with the required data.
An abstract class is a class that comprises the abstract keyword that is present in its declaration. This class can have both abstract and concrete methods. If a user does not write an implementation for a method, it requires them to define that method with the abstract keyword. When a class contains one or more abstract methods, the user has to declare the class with the abstract keyword, which is a non-access modifier in Java.
When a class is abstract, it is not necessary for it to have an abstract method. Users can write almost all the members of a class in an abstract class. You can use abstract classes in various situations. For instance, you can use it if you want to share code with classes that are related to each other closely or if you expect the classes that are extending their abstract class to have several common fields or methods. You can also use it if you wish to declare fields that are both non-static and non-final.
2. Can abstract classes have constructors in Java?
Yes, an abstract class can declare and define a constructor in Java. Since you can not create an instance of an abstract class, a constructor can only be called during constructor chaining, i.e. when you create an instance of the concrete implementation class.
When interviewer ask, what is the purpose of a constructor if you can not instantiate abstract class? Well, it can still be used to initialize common variables, which are declared inside an abstract class, and used by the various implementation.
Also even if you don’t provide any constructor, the compiler will add a default no-argument constructor in an abstract class, without that your subclass will not compile, since the first statement in any constructor implicitly calls super(), default superclass constructor in Java.
3. What is the difference between abstract class and concrete class?
Basically two differences between an abstract class and concrete class. They are:
a) We cannot create an object of abstract class. Only objects of its non-abstract (or concrete) sub classes can be created.
b) It can have zero or more abstract methods that are not allowed in a non-abstract class (concrete class).
All Java versions have their pros and cons, but I really enjoy programming with Java 7. I enjoy the version’s ability to use Java Virtual Machines with non-Java languages. This allows the Java Standard Edition platform to run securely because of the sandbox security model. Although this version remains my favourite, I also use its latest iterations to keep myself updated with new features and use them in my projects.
4. What is Abstract method in Java?
A method which is declared with abstract modifier and has no implementation (means no body) is called abstract method in java.
It does not contain any body. It has simply a signature declaration followed by a semicolon. It has the following general form as given below.
An abstract class may or may not have abstract methods, so it is important for Java users to avoid calling abstract methods in abstract classes. One of the main reasons is that when you call abstract methods, you can create limitations whenever you are implementing those methods. This is because of the initialisation order.
Syntax:
abstract type MethodName(arguments); // No body
For example:
abstract void msg(); // No body.
5.Can you make an instance of an abstract class?
No! You cannot make an instance of an abstract class. An abstract class has to be sub-classed. If you have an abstract class and you want to use a method which has been implemented, you may need to subclass that abstract class, instantiate your subclass and then call that method.
Abstract classes can contain abstract and concrete methods. Abstract classes cannot be instantiated directly i.e. we cannot call the constructor of an abstract class directly nor we can create an instance of an abstract class by using “Class.forName().newInstance()” (Here we get java.lang.InstantiationException). However, if we create an instance of a class that extends an Abstract class, compiler will initialize both the classes. Here compiler will implicitly call the constructor of the Abstract class. Any class that contain an abstract method must be declared “abstract” and abstract methods can have definitions only in child classes.
By overriding and customizing the abstract methods in more than one subclass makes “Polymorphism” and through Inheritance we define body to the abstract methods. Basically an abstract class serves as a template. Abstract class must be extended/subclassed for it to be implemented. A class may be declared abstract even if it has no abstract methods. This prevents it from being instantiated. Abstract class is a class that provides some general functionality but leaves specific implementation to its inheriting classes.
Example of Abstract class:
protected String name;
public String getName() {
return name;
}
public abstract void function();
}
6. In which scenarios do you prefer using an Abstract Class instead of an interface?
Following are the scenarios:
1. When a base class provides default implementations for some methods, while still requiring subclasses to implement specific behavior.
2. When there’s a need to share common state or data among multiple related classes, as abstract classes can have instance variables.
3. When it is necessary to enforce a certain architectural structure within the inheritance hierarchy, abstract classes provide better control.
4. When using languages that do not support multiple inheritance, abstract classes offer an alternative way to reuse code across different classes.
5. When you want to restrict instantiation of a class and only allow its subclasses to be instantiated.
There are so many features that is appreciated in Java, but its large community is what I like most about it. This is a global community where people are creating inventive solutions using Java and sharing their work and experiences through blogs and social media channels. Being a Java user, I get the opportunity to interact with the community, learn from its members and share my projects with them to
7. Can an Abstract Class have both abstract and non-abstract (concrete) methods? Explain your answer with an example.
Yes, an abstract class can have both abstract and non-abstract methods. Abstract classes are designed to provide a common interface for subclasses while allowing partial implementation of their functionality.
For example, consider a Vehicle abstract class with two methods: startEngine() and honk(). The startEngine() method is abstract since the engine starting process may differ among various vehicle types (e.g., cars, motorcycles). However, the honk() method could be concrete as it might share a similar implementation across all vehicles.
public abstract class Vehicle {
public abstract void startEngine();
public void honk() {
System.out.println("Honking!!!");
}
}
public class Car extends Vehicle {
@Override
public void startEngine() {
System.out.println("Starting car engine!!!");
}
}
public class Motorcycle extends Vehicle {
@Override
public void startEngine() {
System.out.println("Starting motorcycle engine!!!");
}
}
In this above example, the Vehicle class has one abstract method (startEngine) and one concrete method (honk), demonstrating that an abstract class can contain both types of methods.
8. Why abstract class has constructor even though you cannot create object?
We cannot create an object of abstract class but we can create an object of subclass of abstract class. When we create an object of subclass of an abstract class, it calls the constructor of subclass.
This subclass constructor has a super keyword in the first line that calls constructor of an abstract class. Thus, the constructors of an abstract class are used from constructor of its subclass.
If the abstract class doesn’t have constructor, a class that extends that abstract class will not get compiled.
9. Why should we create reference to superclass (abstract class reference)?
Create a reference of the superclass to access subclass features because superclass reference allows only to access those features of subclass which have already declared in superclass.
If you create an individual method in subclass, the superclass reference cannot access that method. Thus, any programmer cannot add their own additional features in subclasses other than whatever is given in superclass.
10. What differentiates the interface from the abstract class?
With this question, interviewers aim to assess your fundamental knowledge of the Object Oriented Advanced Business Application Programming (OO ABAP) class. In your answer, note the differences between the interface and abstract class to explain their purposes and components. Focus on their constitution to keep your answer short.
Interfaces are independent structures that you can use in classes to enhance their functionality, which includes methods without implementation. In contrast, an abstract is a distinct type of class that is non-instantiable. Its subclasses are only instantiable when they are not abstract. While interfaces involve methods that exclude implementation, abstract classes are especially for inheritance.
11. When comparing an abstract class with an interface class, which type offers multiple inheritance support and single-instance support and also allows only public members?
You use interfaces in Java when you are expecting unrelated classes to implement your interface. With the help of interfaces, you can specify the behaviour of a data type without concern about who implements the behaviour, along with benefiting from multiple inheritance of type. This helps you define functionality, but not implement it. In the case of an abstract class, it can support single inheritance and even allow users to implement functionality after they have defined it.
When you use abstract classes, you can declare static and final fields. You can also define concrete methods, such as public, protected and private. By default, all fields are public, static and final with interfaces. All the methods that a user defines or declares are also public.
Leave a Reply