Java application architecture
Java application architecture Enterprise OSGi combines two of Java’s most enduringly popular programming models: enterprise Java and OSGi. Enterprise Java is a loosely defined set of libraries, APIs, and frameworks built on top of core Java that turn it into a powerful platform for distributed, transactional, interactive, and persistent applications. Enterprise Java has been hugely successful, but as the scale and complexity of enterprise Java applications have grown, they’ve started to look creaky, bloated, and monolithic. OSGi applications, on the other hand, tend to be compact, modular, and maintainable. But the OSGi programming model is pretty low-level.
It doesn’t have much to say about transactions, persistence, or web pages, all of which are essential underpinnings for many modern Java programs. What about a combination, something with the best features of both enterprise Java and OSGi? Such a programming model would enable applications that are modular, maintainable, and take advantage of industry standard enterprise Java libraries. Until recently, this combination was almost impossible, because enterprise Java and OSGi didn’t work together. Now they do, and we hope you’ll agree with us that the merger is pretty exciting.
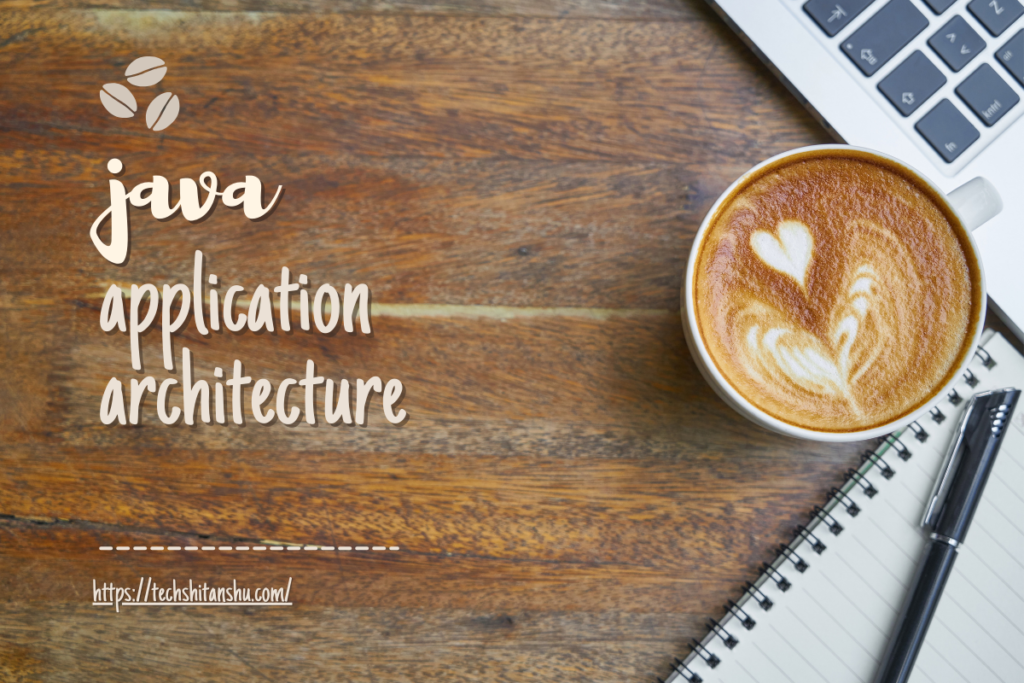
Class Method Area: This memory region stores class-level data during code execution. It holds information such as static variables, static methods, static blocks, and instance methods.
Heap: The heap is a segment of JVM memory created upon JVM startup. Its size is dynamic, adjusting up or down as the application runs.
Stack: Also known as the thread stack, it is allocated for each execution thread. The stack is used to store partial results, local variables, and data related to method calls and returns.
Native Stack: This area contains information about all native methods used in the application.
Execution Engine: The core component of the JVM, responsible for executing bytecode and Java classes. It consists of three main components:
-
Interpreter: Converts bytecode into native code and executes it sequentially. It processes code one instruction at a time and can interpret the same method multiple times, which can impact performance. To address this, the JIT compiler is utilized.
-
JIT Compiler: The Just-In-Time (JIT) compiler improves performance by compiling bytecode into native code before execution, thus enhancing execution speed and overall system performance.
-
Garbage Collector: Manages memory by identifying and reclaiming unused memory. It operates in two phases: Mark and Sweep. During the Mark phase, it identifies used and unused memory chunks. In the Sweep phase, it removes the objects identified during Mark.
Java Native Interface (JNI): Acts as a bridge between Java method calls and native libraries, facilitating interaction with native code.
Object-Oriented Programming
Object-oriented programming (OOP) is at the core of Java. In fact, all Java programs are to at least some extent object-oriented. OOP is so integral to Java that it is best to understand its basic principles before you begin writing even simple Java programs. Therefore, this begins with a discussion of the theoretical aspects of OOP.
Two Paradigms
All computer programs consist of two elements: code and data. Furthermore, a program can be conceptually organized around its code or around its data. That is, some programs are written around “what is happening” and others are written around “who is being affected.” These are the two paradigms that govern how a program is constructed. The first way is called the process-oriented model. This approach characterizes a program as a series of
linear steps (that is, code). The process-oriented model can be thought of as code acting on data. Procedural languages such as C employ this model to considerable success. However, as mentioned in, problems with this approach appear as programs grow larger
and more complex.
To manage increasing complexity, the second approach, called object-oriented programming, was conceived. Object-oriented programming organizes a program around its data (that is, objects) and a set of well-defined interfaces to that data. An object-oriented program can be characterized as data controlling access to code. As you will see, by switching the controlling entity to data, you can achieve several organizational benefits.
An essential element of object-oriented programming is abstraction. Humans manage complexity through abstraction. For example, people do not think of a car as a set of tens of thousands of individual parts. They think of it as a well-defined object with its own unique behavior. This abstraction allows people to use a car to drive to the grocery store without being overwhelmed by the complexity of the parts that form the car. They can ignore the details of how the engine, transmission, and braking systems work. Instead, they are free to utilize the object as a whole.
A powerful way to manage abstraction is through the use of hierarchical classifications. This allows you to layer the semantics of complex systems, breaking them into more manageable pieces. From the outside, the car is a single object. Once inside, you see that the car consists of several subsystems: steering, brakes, sound system, seat belts, heating, cellular phone, and so on. In turn, each of these subsystems is made up of more specialized units. For instance, the sound system consists of a radio, a CD player, and/or a tape or MP3 player. The point is that you manage the complexity of the car (or any other complex system) through the use of hierarchical abstractions.
Hierarchical abstractions of complex systems can also be applied to computer programs. The data from a traditional process-oriented program can be transformed by abstraction into its component objects. A sequence of process steps can become a collection of messages between these objects. Thus, each of these objects describes its own unique behavior. You can treat these objects as concrete entities that respond to messages telling them to do
something. This is the essence of object-oriented programming. Object-oriented concepts form the heart of Java just as they form the basis for human
understanding. It is important that you understand how these concepts translate into programs.
As you will see, object-oriented programming is a powerful and natural paradigm for creating programs that survive the inevitable changes accompanying the life cycle of any major software project, including conception, growth, and aging. For example, once you have well-defined objects and clean, reliable interfaces to those objects, you can gracefully decommission or replace parts of an older system without fear.
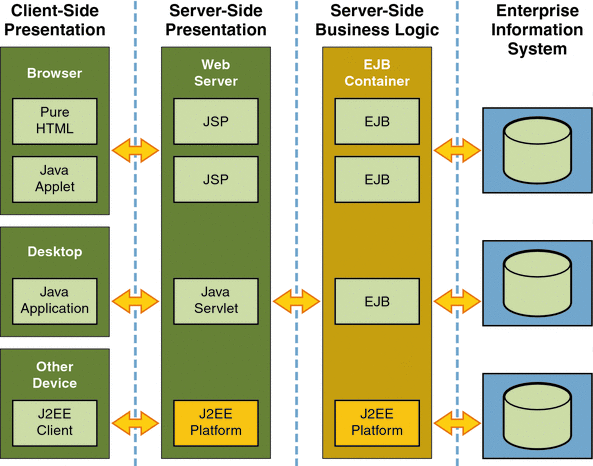
Developers today increasingly recognize the need for distributed, transactional, and portable applications that leverage the speed, security, and reliability of server-side technology. Enterprise applications provide the business logic for an enterprise. They are centrally managed and often interact with other enterprise software. In the world of information technology, enterprise applications must be designed, built, and produced for less money, with greater speed, and with fewer resources.
With the Platform, Enterprise Edition ( EE), development of enterprise applications has never been easier or faster. The aim of the EE platform is to provide developers with a powerful set of APIs while shortening development time, reducing application complexity, and improving application performance.
The EE platform is developed through the Community Process (JCP), which is responsible for all technologies. Expert groups composed of interested parties have created Specification Requests (JSRs) to define the various EE technologies. The work of the Community under the JCP program helps to ensure technology’s standards of stability and cross-platform compatibility.
The EE platform uses a simplified programming model. XML deployment descriptors are optional. Instead, a developer can simply enter the information as an annotation directly into a source file, and the EE server will configure the component at deployment and runtime. These annotations are generally used to embed in a program data that would otherwise be furnished in a deployment descriptor. With annotations, you put the specification information in your code next to the program element affected.
In the EE platform, dependency injection can be applied to all resources a component needs, effectively hiding the creation and lookup of resources from application code. Dependency injection can be used in Enterprise Beans (EJB) containers, web containers, and application clients. Dependency injection allows the EE container to automatically insert references to other required components or resources, using annotations.
This tutorial uses examples to describe the features available in the EE platform for developing enterprise applications. Whether you are a new or experienced enterprise developer, you should find the examples and accompanying text a valuable and accessible knowledge base for creating your own solutions.
Leave a Reply