Java Lambda Expressions Interview Questions and Answers
Added by JDK 8, lambda expressions (and their related features) significantly enhance Java because of two primary reasons. First, they add new syntax elements that increase the expressive power of the language. In the process, they streamline the way that certain common constructs are implemented. Second, the addition of lambda expressions resulted in new capabilities being incorporated into the API library.
Among these new capabilities are the ability to more easily take advantage of the parallel processing capabilities of multi-core environments, especially as it relates to the handling of for-each style operations, and the new stream API, which supports pipeline operations on data. The addition of lambda expressions also provided the catalyst for other new Java features, including the default method, which lets you define default behaviour for an interface method, and the method reference , which lets you refer to a method without executing it.
TechShitanshu has prepared a list of the top java Abstract Class interview questions and answers that are frequently asked in the interview. It is going to help you to crack the java interview questions and answers to get your dream job.
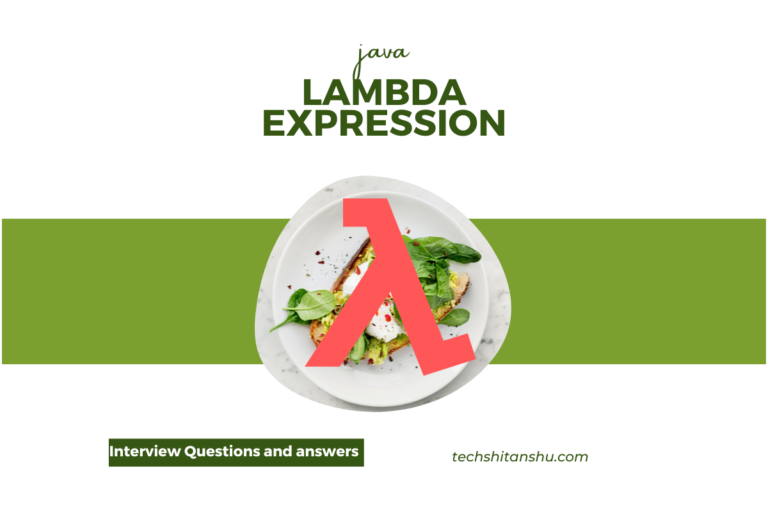
Java Lambda Expressions Interview Questions and Answers
1. What is lambda expression of Java 8?
In Java the lambda expression introduces a new syntax element and operator into the Java language. The new operator, sometimes referred to as the lambda operator or the arrow operator, is −>. It divides a lambda expression into two parts. The left side specifies any parameters required by the lambda expression. (If no parameters are needed, an empty parameter list is used.) On the right side is the lambda body, which specifies the actions of the lambda expression.
The −> can be verbalized as “becomes” or “goes to.” Java defines two types of lambda bodies. One consists of a single expression, and the other type consists of a block of code. We will begin with lambdas that define a single expression. Lambdas with block bodies are discussed later in this chapter.
At this point, it will be helpful to look a few examples of lambda expressions before continuing. Let’s begin with what is probably the simplest type of lambda expression youcan write.
It evaluates to a constant value and is shown here:
() -> 123.45
This lambda expression takes no parameters, thus the parameter list is empty. It returns the constant value 123.45. Therefore, it is similar to the following method:
double myMeth() { return 123.45; }
Of course, the method defined by a lambda expression does not have a name. A slightly more interesting lambda expression is shown here:
() -> Math.random() * 100
This lambda expression obtains a pseudo-random value from Math.random( ), multiplies it by 100, and returns the result. It, too, does not require a parameter. When a lambda expression requires a parameter, it is specified in the parameter list on the left side of the lambda operator. Here is a simple example:
(n) -> (n % 2)==0
This lambda expression returns true if the value of parameter n is even. Although it is possible to explicitly specify the type of a parameter, such as n in this case, often you won’t need to do so because in many cases its type can be inferred. Like a named method, a lambda expression can specify as many parameters as needed.
2. Why to use Lambda Expression?
Readability: By reducing boilerplate code, lambda expressions can make the main logic of a program more apparent. The concise syntax allows for clear expression of the computation or action being performed.
3. Can you pass lambda expression to a method? And When?
Yes, we can pass a lambda expression to a method provided it is expecting a functional interface. For example, if a method is accepting a Runnable, Comparable or Comparator then you can pass a lambda expression to it because all these are functional interface in Java 8.
4. What is functional interface in Java?
As stated, a functional interface is an interface that specifies only one abstract method. If you have been programming in Java for some time, you might at first think that all interface methods are implicitly abstract. Although this was true prior to JDK 8, the situation has changed. As explained in Chapter 9, beginning with JDK 8, it is possible to specify default behaviour for a method declared in an interface. This is called a default method. Today, an interface method is abstract only if it does not specify a default implementation. Because nondefault interface methods are implicitly abstract, there is no need to use the abstract modifier (although you can specify it, if you like).
Here is an example of a functional interface:
interface MyNumber {
double getValue();
}
5. Can a functional interface extend/inherit another interface?
A functional interface cannot extend another interface with abstract methods as it will void the rule of one abstract method per functional interface.
interface Parent {
public int parentMethod();
}
@FunctionalInterface // This cannot be FunctionalInterface
interface Child extends Parent {
public int childMethod();
// It will also extend the abstract method of the Parent Interface
// Hence it will have more than one abstract method
// And will give a compiler error
}
It can extend other interfaces which do not have any abstract method and only have the default, static, another class is overridden, and normal methods. For eg.
interface Parent {
public void parentMethod(){
System.out.println("Hello");
}
}
@FunctionalInterface
interface Child extends Parent {
public int childMethod();
}
6. Explain some standard Java pre-defined functional interfaces?
Some of the famous pre-defined functional interfaces from previous Java versions are Runnable, Callable, Comparator, and Comparable. While Java 8 introduces functional interfaces like Supplier, Consumer, Predicate, etc. Please refer to the java.util.function doc for other predefined functional interfaces and its description introduced in Java 8.
Runnable: use to execute the instances of a class over another thread with no arguments and no return value.
Callable: use to execute the instances of a class over another thread with no arguments and it either returns a value or throws an exception.
Comparator: use to sort different objects in a user-defined order
Comparable: use to sort objects in the natural sort order
7. What before Java 8 code does, and Lambda expression simplify?
Lambda expression simplifies the inner class and anonymous inner class code, which usually suffer from the ‘vertical’ problem (Too many lines of code required to implement a basic logic). Lambda expressions avoid the ‘vertical’ problem by simplifying and reducing the number of lines required to implement the inner class functionality.
8. Can we use lambda expressions to implement interfaces having default and static methods?
Lambda expressions can be used implement interfaces having default and static methods only if there is a single abstract method in the interface. This called a functional interface.
From Java 8 onwards, an interface can contain default methods and static methods whose implementation is defined directly in the interface declaration.
9. How many parameters a lambda expression have?
A lambda expression can have zero, one or multiple parameters.
//Zero parameter lambda expression
() -> System.out.println('No parameters');
//One parameter lambda expression
(i) -> i*10;
//Multiple parameter lambda expression
(i1, i1) -> System.out.println(i1+i2);
10. Describe Some of the Functional Interfaces in the Standard Library.
There are a lot of functional interfaces in the java.util.function package. The more common ones include, but are not limited to:
- Function – it takes one argument and returns a result
- Consumer – it takes one argument and returns no result (represents a side effect)
- Supplier – it takes no arguments and returns a result
- Predicate – it takes one argument and returns a boolean
- BiFunction – it takes two arguments and returns a result
- BinaryOperator – it is similar to a BiFunction, taking two arguments and returning a result. The two arguments and the result are all of the same types.
- UnaryOperator – it is similar to a Function, taking a single argument and returning a result of the same type
Leave a Reply