Java Method Overloading and Overriding Top 30 Interview Questions and Answers
Java is somewhat unusual in that it allows two different subroutines in the same class to have the same name, provided that their signatures are different. (The language C++ on which Java is based also has this feature.) When this happens, we say that the name of the subroutine is overloaded because it has several different meanings.
When you write a method in a subclass that has the same signature as a method in its superclass, the method from the superclass is hidden in the same way. We say that the method in the subclass overrides the method from the superclass. Again, however, super can be used to access the method from the superclass.
TechShitanshu has prepared a list of the top java method overloading and overriding interview questions and answers that are frequently asked in the interview. It is going to help you to crack the java interview questions to get your dream job.
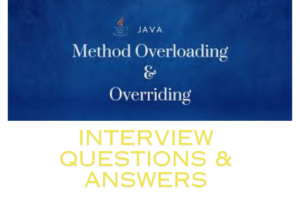
Java Method Overloading and Overriding Interview Questions and Answers
Table of Contents
1. What is the other name of Java Method Overloading and Overriding?
Java Method Overloading is also known as Static Polymorphism and it is the most basic interview question for java method overloading and overriding
2. What is method overloading in Java?
3. What is method overriding in Java?
While inheriting a superclass, if a subclass has the same instance method with the same method signature as that of the super class’s method then it is said to be method overriding in java.
Reason to have another exactly same method in inheriting class is to provide more specific implementation from that of more general implementation in a superclass
4. The order of the formal parameters (inputs) of an overriding method in a subclass can be changed or not?
No, number of formal parameters and their order should be exactly the same as that of the super class’s overridden method. java method overloading and overriding typical java interview questions
5. What are the rules of overloading a method in Java?
1. Method Signature
The first and foremost rule to overload a method in Java is to change the method signature. the method signature is made of a number of arguments, types of arguments, and order of arguments if they are of different types.
You can change any of these or combinations of them to overload a method in Java. For example, in the following code, we have changed a number of arguments to overload whoAmI() method in Java :
class UNIX { protected final void whoAmI() { System.out.println(“I am UNIX”); } protected final void whoAmI(String name){ System.out.println(“I am ” + name); } }
By the way, the right way to overload a method is to change the number of arguments or types of arguments because it denotes that it offers the same functionality but the input is different.
For example, the println() method of PrintStream class has several overloaded versions to accept different data types like String, int, long, float, double, boolean, etc.
Similarly, the EnumSet.of() method is overloaded to accept one, two, or more values. The third way to overload method by changing the order of argument or type of argument creates a lot of confusion and best to be avoided, as discussed in my post about overloading best practices in Java.
2. Method Return Type
The return type of method is not part of the method signature, so just changing the return type will not overload a method in Java. In fact, just changing the return type will result in a compile-time error as “duplicate method X in type Y.
6. What are the rules of overloading a method in Java?
Method Overriding Rules in Java:
Overriding is completely different than overloading and so its rules are also different. For terminology, the original method is known as overridden method and the new method is known as the overriding method. Following rules must be followed to correctly override a method in Java interview questions :
1. Location
A method can only be overridden in sub-class, not in the same class. If you try to create two methods with the same signature in one class compiler will complain about it saying “duplicate method in type Class”, as shown in the following screenshot :
2. Exception
Overriding method cannot throw checked Exception which is higher in the hierarchy, than checked Exception thrown by the overridden method.
For example, if an overridden method throws IOException or ClassNotfoundException, which are checked Exception then the overriding method can not throw java.lang.Exception because it comes higher in type hierarchy (it’s the superclass of IOException and ClassNotFoundExcepiton).
If you do so, the compiler will catch.
3. Visibility
The overriding method can not reduce access of overridden method. It means if the overridden method is defined as public then the overriding method can not be protected or package-private.
Similarly, if the original method is protected then the overriding method cannot be package-private.
You can see what happens if you violate this rule in Java, as seen in this screenshot it will throw compile time error saying “You cannot reduce the visibility of inherited method of a class”.
4. Accessibility
Overriding method can increase access of overridden method. This is the opposite of the earlier rule, according to this if the overridden method is declared as protected then the overriding method can be protected or public.
5. Types of Methods
The private, static, and final methods can not be overridden in Java. See other articles in this blog to learn why you cannot override private, static, or final methods in Java.
By the way, you can hide private and static methods but trying to override the final method will result in compile-time error “Cannot override the final method from a class”.
6. Return Type
The return type of overriding method must be the same as overridden method. Trying to change the return type of method in the child class will throw compile-time error “return type is incompatible with parent class method” as shown in the following screenshot.
That’s all on Rules of method overloading and overriding in Java, It’s very important to remember these rules to correctly overload and override any method in Java. Also remember to use @Override annotation to accidentally overloading a method, instead of overriding it.
7. Can we change the access level of the overriding method in a subclass?
While overriding super class’s method to a subclass, access level can be kept same or it should be wider/broader i.e. to increase the access visibility of the overriding method in a subclass.
8. Can we reduce the visibility of the inherited or overridden method?
No, we can either need to stick with the same access level or else it can widen to upper access level
9. Whether class compiles successfully if we decrease the access visibility of the overriding method in a subclass?
- No, a compile-time error will be thrown
- Access level should be wider/broader but we can’t decrease the access visibility of the overriding method in a subclass
- Access level increases in below order (with private being the least and public being the highest)
private–> default –> protected –> public
Leave a Reply