The New Features Of Java 17 : Java Development Kit (JDK) 17
This blog describes some of the enhancements in Java SE 17 and JDK 17. In some cases, the descriptions provide links to additional detailed information about an issue or a change. The APIs described here are provided with the Oracle JDK. It includes a complete implementation of the Java SE 17 Platform and additional Java APIs to support developing, debugging, and monitoring Java applications. Another source of information about important enhancements and new features in Java SE 17 and JDK 17 is the Java SE 17 ( JSR 392)
Platform Specification, which documents the changes to the specification made between Java SE 16 and Java SE 17. This document includes descriptions of those new features and enhancements that are also changes to the specification. The descriptions also identify potential compatibility issues that you might encounter when migrating to JDK 17.
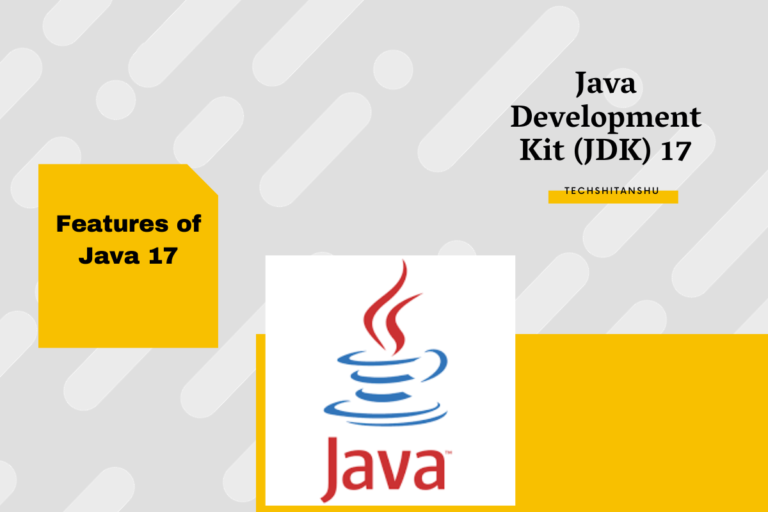
Sealed Classes
Sealed Classes have been added to the Java Language as new features of java 17. Sealed classes and interfaces restrict which other classes or interfaces may extend or implement them.
Sealed Classes were proposed by JEP 360 and delivered in JDK 15 as a preview feature. They were proposed again, with refinements, by JEP 397 and delivered in JDK 16 as a preview feature. Now in JDK 17, Sealed Classes are being finalized with no changes from JDK 16.
Pattern Matching for switch (Preview)
Enhance the Java programming language with pattern matching for switch expressions and statements, along with extensions to the language of patterns. Extending pattern matching to switch allows an expression to be tested against a number of patterns, each with a specific action, so that complex data-oriented queries can be expressed concisely and safely.
New macOS Rendering Pipeline
The Java 2D API used by the Swing APIs for rendering, can now use the new Apple Metal accelerated rendering API for macOS. This is currently disabled by default, so rendering still uses OpenGL APIs, which are deprecated by Apple but still available and supported.
To enable Metal, an application should specify its use by setting the system property:
-Dsun.java2d.metal=true
Use of Metal or OpenGL is transparent to applications since this is a difference of internal implementation and has no effect on Java APIs. The metal pipeline requires macOS 10.14.x or later. Attempts to set it on earlier releases will be ignored.
New API for Accessing Large Icons
A new method in features of java 17, javax.swing.filechooser.FileSystemView.getSystemIcon(File, int, int)
, is available in JDK 17 that enables access to higher quality icons when possible. It is fully implemented for the Windows platform; however, results on other platforms might vary and will be enhanced later. For example, by using the following code:
FileSystemView fsv = FileSystemView.getFileSystemView();
Icon icon = fsv.getSystemIcon(new File("application.exe"), 64, 64);
JLabel label = new JLabel(icon);
The user can obtain a higher quality icon for the “application.exe” file. This icon is suitable for creating a label that can be better scaled in a HighDPI environment.
DatagramSocket Can Be Used Directly to Join Multicast Groups
java.net.DatagramSocket
has been updated in this release to add support for joining multicast groups. It now defines joinGroup
and leaveGroup
methods to join and leave multicast groups. The class level API documentation of java.net.DatagramSocket
has been updated to explain how a plain DatagramSocket
can be configured and used to join and leave multicast groups.
This change means that the DatagramSocket
API can be used for multicast applications without needing to use the legacy java.net.MulticastSocket
API. The MulticastSocket
API works as before, although most of its methods are deprecated.
More information on the rationale of this change can be seen in the CSR JDK-8260667.
Add support for UserDefinedFileAttributeView on macOS
The file system provider implementation on macOS has been updated in this release to support extended attributes. The java.nio.file.attribute.UserDefinedFileAttributeView
API can now be used to obtain a view of a file’s extended attributes. This (optional) view was not supported in previous JDK releases.
JEP 356: Enhanced Pseudo-Random Number Generators
Provide new interface types and implementations for pseudorandom number generators (PRNGs), including jumpable PRNGs and an additional class of splittable PRNG algorithms (LXM).
For further details, see JEP 356.
Modernization of Ideal Graph Visualizer
Ideal Graph Visualizer (IGV), a tool to explore visually and interactively the intermediate representation used in the HotSpot VM C2 just-in-time (JIT) compiler, has been modernized. Enhancements include:
- Support for running IGV on up to JDK 15 (the latest version supported by IGV’s underlying NetBeans Platform)
- Faster, Maven-based IGV build system
- Stabilization of block formation, group removal, and node tracking
- More intuitive coloring and node categorization in default filters
- Ranked quick node search with more natural default behavior
The modernized IGV is partially compatible with graphs generated from earlier JDK releases. It supports basic functionality such as graph loading and visualization, but auxiliary functionality such as node clustering and coloring might be affected.
Details about building and running IGV are available in the src/utils/IdealGraphVisualizer/README.md
file in the tool’s source directory and a snippet below:
The JVM support is controlled by the flag -XX:PrintIdealGraphLevel=# where # is:
0: no output, the default
1: dumps graph after parsing, before matching, and final code (also dumps graphs for failed compilations, if available)
2: more detail, including after loop opts
3: even more detail
4: prints graph after parsing every bytecode (very slow)
By default the JVM expects that it will connect to a visualizer on the local host on port 4444. This can be configured using the options -XX:PrintIdealGraphAddress= and -XX:PrintIdealGraphPort=. PrintIdealGraphAddress can actually be a hostname.
It is advisable to run the JVM with background compilation disabled (-Xbatch). Compilations going on in the background may be cancelled when the VM terminates, which can lead to incomplete dumps being sent to IGV.
Alternatively the output can be sent to a file using -XX:PrintIdealGraphFile=filename. Each compiler thread will get it's own file with unique names being generated by adding a number onto the provided file name.
Source Details in Error Messages
When JavaDoc reports an issue in an input source file, it displays the source line for the issue, and a line containing a caret (^
) pointing to the position on the line, in a manner similar to compiler (javac
) diagnostic messages.
In addition, logging and other “info” messages are now written to the standard error stream, leaving the standard output stream to be used for output that is specifically requested by command-line options, such as command-line help.
New Page for "New API" and Improved "Deprecated" Page
JavaDoc can now generate a page summarizing the recent changes in an API. The list of recent releases to be included is specified with the --since
command-line option. These values are used to find the declarations with matching @since
tags to be included on the new page. The --since-label
command-line option provides text to use in the heading of the “New API” page.
On the page that summarizes deprecated items, you can view items grouped by the release in which they were deprecated.
JDK Flight Recorder Event for Deserialization
It is now possible to monitor deserialization of objects using JDK Flight Recorder (JFR). When JFR is enabled and the JFR configuration includes deserialization events, JFR will emit an event whenever the running program attempts to deserialize an object. The deserialization event is named jdk.Deserialization
, and it is disabled by default. The deserialization event contains information that is used by the serialization filter mechanism; see the ObjectInputFilter specification. Additionally, if a filter is enabled, the JFR event indicates whether the filter accepted or rejected deserialization of the object.
Implement Context-Specific Deserialization Filters
JEP 415: Context-Specific Deserialization Filters allows applications to configure context-specific and dynamically-selected deserialization filters via a JVM-wide filter factory that is invoked to select a filter for each individual deserialization operation.
The Java Core Libraries Developers Guide for Serialization Filtering describes use cases and provides examples.
System Property for Native Character Encoding Name
A new system property native.encoding
has been introduced. This system property provides the underlying host environment’s character encoding name. For example, typically it has UTF-8
in Linux and macOS platforms, and Cp1252
in Windows (en-US). Refer to the CSR for more detail.
Foreign Function & Memory API (Incubator)
java.io.Console
has been updated to define a new method that returns the Charset
for the console. The returned Charset may be different from the one returned from Charset.defaultCharset()
method. For example, it returns IBM437
while Charset.defaultCharset()
returns windows-1252
on Windows (en-US). Refer to the CSR for more detail.
Console Charset API
Introduce an API by which Java programs can interoperate with code and data outside of the Java runtime. By efficiently invoking foreign functions (i.e., code outside the JVM), and by safely accessing foreign memory (i.e., memory not managed by the JVM), the API enables Java programs to call native libraries and process native data without the brittleness and danger of JNI.
For further details, see JEP 412.
Add java.time.InstantSource
A new interface java.time.InstantSource
has been introduced. This interface is an abstraction from java.time.Clock
that only focuses on the current instant and does not refer to the time zone.
Hex Formatting and Parsing Utility
java.util.HexFormat
provides conversions to and from hexadecimal for primitive types and byte arrays. The options for delimiter, prefix, suffix, and uppercase or lowercase are provided by factory methods returning HexFormat instances.
Experimental Compiler Blackholes Support
The experimental support for Compiler Blackholes is added. These are useful for low-level benchmarking, to avoid dead-code elimination on the critical paths, without affecting the benchmark performance. Current support is implemented as CompileCommand, accessible as -XX:CompileCommand=blackhole,<method>
, with the plan to eventually graduate it to a public API.
JMH is already able to auto-detect and use this facility when instructed/available. Please consult JMH documentation for the next steps.
New Class Hierarchy Analysis Implementation in the HotSpot JVM
A new Class Hierarchy Analysis implementation is introduced in the HotSpot JVM. It features enhanced handling of abstract and default methods which improves inlining decisions made by the JIT-compilers. The new implementation supersedes the original one and is turned on by default.
To help diagnose possible issues related to the new implementation, the original implementation can be turned on by specifying the -XX:+UnlockDiagnosticVMOptions -XX:-UseVtableBasedCHA
command-line flags.
The original implementation may be removed in a future release.
JEP 391: macOS/AArch64 Port
macOS 11.0 now supports the AArch64 architecture. This JEP implements support for the macos-aarch64 platform in the JDK. One of the features added is support for the W^X (write xor execute) memory. It is enabled only for macos-aarch64 and can be extended to other platforms at some point. The JDK can be either cross-compiled on an Intel machine or compiled on an Apple M1-based machine.
For further details, see JEP 391.
Unified Logging Supports Asynchronous Log Flushing
To avoid undesirable delays in a thread using unified logging, the user can now request that the unified logging system operate in asynchronous mode. This is done by passing the command-line option -Xlog:async
. In asynchronous logging mode, log sites enqueue all logging messages to a buffer. A standalone thread is responsible for flushing them to the corresponding outputs. The intermediate buffer is bounded. On buffer exhaustion, the enqueuing message is discarded. The user can control the size of the intermediate buffer by using the command-line option -XX:AsyncLogBufferSize=<bytes>
.
macOS on ARM Early Access Available
A new macOS is now available for ARM systems. The ARM port should behave similarly to the Intel port. There are no known feature differences. When reporting issues on macOS, please specify if using ARM or x64.
Provide Support for Specifying a Signer in Keytool -genkeypair Command
The -signer
and -signerkeypass
options have been added to the -genkeypair
command of the keytool
utility. The -signer
option specifies the keystore alias of a PrivateKeyEntry
for the signer and the -signerkeypass
option specifies the password used to protect the signer’s private key. These options allow keytool -genkeypair
to sign the certificate by using the signer’s private key. This is especially useful for generating a certificate with a key agreement algorithm as its public key algorithm.
SunJCE Provider Supports KW and KWP Modes With AES Cipher
The SunJCE provider has been enhanced to support the AES Key Wrap Algorithm (RFC 3394) and the AES Key Wrap with Padding Algorithm (RFC 5649). In earlier releases, the SunJCE provider supported RFC 3394 under the “AESWrap” cipher algorithm that could only be used to wrap and unwrap keys. With this enhancement, two block cipher modes, KW and KWP, have been added that support data encryption/decryption and key wrap/unwrap by using AES. Please check the “SunJCE provider” section of the “JDK Providers Documentation” guide for more details.
New SunPKCS11 configuration properties
SunPKCS11 provider adds new provider configuration attributes to better control native resources usage. The SunPKCS11 provider consumes native resources in order to work with native PKCS11 libraries. To manage and better control the native resources, additional configuration attributes are added to control the frequency of clearing native references as well as whether to destroy underlying PKCS11 Token after logout.
The 3 new attributes for SunPKCS11 provider configuration file are:
destroyTokenAfterLogout
(boolean, defaults to false) If set to true, whenjava.security.AuthProvider.logout()
is called upon the SunPKCS11 provider instance, the underlying Token object will be destroyed and resources will be freed. This essentially renders the SunPKCS11 provider instance unusable after logout() calls. Note that a PKCS11 provider with this attribute set to true should not be added to the system provider list since the provider object is not usable after a logout() method call.cleaner.shortInterval
(integer, defaults to 2000, in milliseconds) This defines the frequency for clearing native references during busy period, i.e. how often should the cleaner thread processes the no-longer-needed native references in the queue to free up native memory. Note that the cleaner thread will switch to the ‘longInterval’ frequency after 200 failed tries, i.e. when no references are found in the queue.cleaner.longInterval
(integer, defaults to 60000, in milliseconds) This defines the frequency for checking native reference during non-busy period, i.e. how often should the cleaner thread check the queue for native references. Note that the cleaner thread will switch back to the ‘shortInterval’ value if native PKCS11 references for cleaning are detected.
SunPKCS11 Provider Supports ChaCha20-Poly1305 Cipher and ChaCha20 KeyGenerator if Supported by PKCS11 Library
SunPKCS11 provider is enhanced to support the following crypto services and algorithms when the underlying PKCS11 library supports the corresponding PKCS#11 mechanisms:
- ChaCha20 KeyGenerator <=> CKM_CHACHA20_KEY_GEN mechanism
- CHACHA20-POLY1305 Cipher <=> CKM_CHACHA20_POLY1305 mechanism
- CHACHA20-POLY1305 AlgorithmParameters <=> CKM_CHACHA20_POLY1305 mechanism
- CHACHA20 SecretKeyFactory <=> CKM_CHACHA20_POLY1305 mechanism
Configurable Extensions With System Properties
Two new system properties have been added. The system property, jdk.tls.client.disableExtensions
, is used to disable TLS extensions used in the client. The system property, jdk.tls.server.disableExtensions
, is used to disable TLS extensions used in the server. If an extension is disabled, it will be neither produced nor processed in the handshake messages.
The property string is a list of comma separated standard TLS extension names, as registered in the IANA documentation (for example, server_name, status_request, and signature_algorithms_cert). Note that the extension names are case sensitive. Unknown, unsupported, misspelled and duplicated TLS extension name tokens will be ignored.
Please note that the impact of blocking TLS extensions is complicated. For example, a TLS connection may not be able to be established if a mandatory extension is disabled. Please do not disable mandatory extensions, and do not use this feature unless you clearly understand the impact.
Use permitted_enctypes if default_tkt_enctypes or default_tgs_enctypes is not present
Use permitted_enctypes as the default value of default_tkt_enctypes or default_tgs_enctypes if any of the them are not defined in krb5.conf.
"Related Packages" on a Package Summary Page
The summary page for a package now includes a section listing any “related packages”. The set of related packages is determined heuristically on common naming conventions, and may include the following:
- The “parent” package (that is, the package for which a package is a subpackage)
- Sibling packages (that is, other packages with the same parent package)
- Any subpackages
The related packages need not all be in the same module.
Pingback: Java Sealed Classes in JDK 17 - TECH SHITANSHU
Pingback: Java Pattern Matching Elegance in JDK17 - TECH SHITANSHU